[목차]
01. include로 화면 구현
💡
회원전체조회 리스트 화면에 include로 회원가입 폼과 연결한다.
➡️ 회원가입 화면 + 리스트 화면
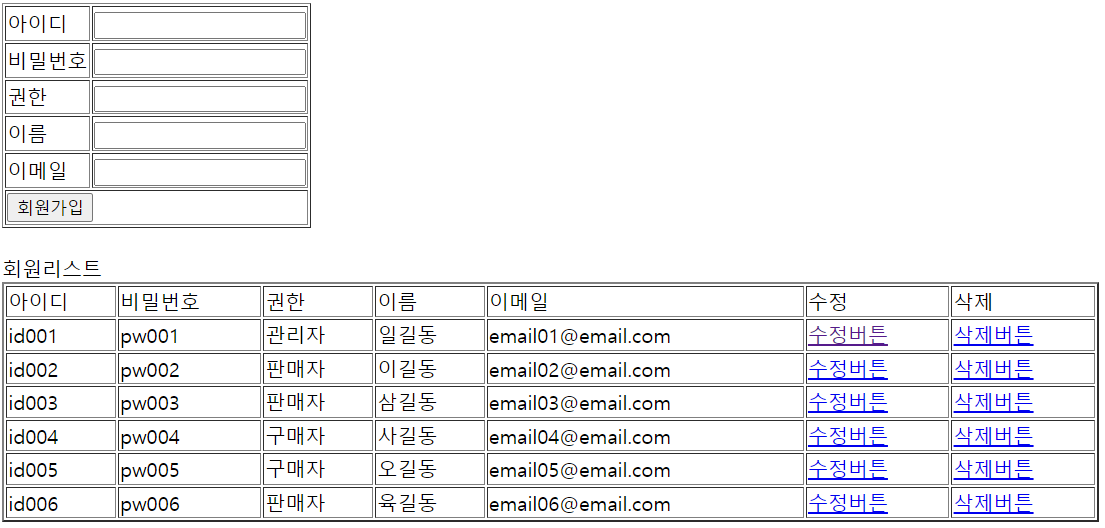
[코드예제] user_list.jsp
<%@ page language="java" contentType="text/html; charset=EUC-KR" pageEncoding="EUC-KR"%> <!DOCTYPE html> <%@ page import = "java.sql.DriverManager" %> <%@ page import = "java.sql.Connection" %> <%@ page import = "java.sql.PreparedStatement" %> <%@ page import = "java.sql.ResultSet" %> <%@ page import = "java.sql.SQLException" %> <%@ include file = "/uinsert/user_insert_form.jsp" %> <br/> 회원리스트 <br/> <table width="100%" border="2"> <tr> <td>아이디</td> <td>비밀번호</td> <td>권한</td> <td>이름</td> <td>이메일</td> <td>수정</td> <td>삭제</td> </tr> <% // 1단계 : 드라이버 로딩 Class.forName("com.mysql.jdbc.Driver"); Connection conn = null; PreparedStatement pstmt = null; ResultSet rs = null; try { // 2단계 : DB연결 String jdbcDriver = "jdbc:mysql://localhost:3306/dbhooni?" + "useUnicode=true&characterEncoding=euckr"; String dbUser = "idhooni"; String dbPass = "pwhooni"; conn = DriverManager.getConnection(jdbcDriver, dbUser, dbPass); // 3단계 : 쿼리 실행을 위한 객체 생성 pstmt = conn.prepareStatement("SELECT * FROM tb_user"); // 4단계 : 쿼리 실행 rs = pstmt.executeQuery(); // System.out.println(rs.next()); // 5단계 : 쿼리 실행 결과 사용 while(rs.next()) { //System.out.println("반복횟수"); %> <tr> <td><%= rs.getString("u_id") %></td> <td><%= rs.getString("u_pw") %></td> <td><%= rs.getString("u_level") %></td> <td><%= rs.getString("u_name") %></td> <td><%= rs.getString("u_email") %></td> <td><a href="<%= request.getContextPath() %>/userupdate/user_update_form.jsp?send_id=<%= rs.getString("u_id") %>">수정버튼</a></td> <td><a href="<%= request.getContextPath() %>/userdelete/user_delete_action.jsp?send_id=<%= rs.getString("u_id") %>">삭제버튼</a></td> </tr> <% } } catch(SQLException ex) { out.println(ex.getMessage()); ex.printStackTrace(); } finally { // 6. 사용한 Statement 종료 if (rs != null) try { rs.close(); } catch(SQLException ex) {} if (pstmt != null) try { pstmt.close(); } catch(SQLException ex) {} // 7. 커넥션 종료 if (conn != null) try { conn.close(); } catch(SQLException ex) {} } %> </table>
02. redirect로 화면 연결
💡
회원가입, 수정, 삭제 후 화면 처리를 user_list.jsp 로 리다이렉트 한다.
[코드예제] user_(insert, update, delete)_action.jsp
// 각각 action.jsp 자바코드 맨 마지막 부분에 리다이렉트 코드 추가 // user_list.jsp로 리다이렉트 response.sendRedirect(request.getContextPath() + "/ulist/user_list.jsp");
03. 검색 조건 설정
💡
1️⃣ 선택 후 입력하는 폼 만들기 (select - option 사용)
<jdbc 프로그램 실행>
2️⃣ 객체 생성 단계에서 select 쿼리 사용
3️⃣ 조건 설정 (null 2개, null 1개, null 0개 각각 select 쿼리 객체 생성)
4️⃣ null 0개 시 조건 설정 (id, level, name, eamil 일때 - WHERE 조건)
5️⃣ 쿼리 실행결과 사용
[코드예제] user_search_form.jsp
<%@ page language="java" contentType="text/html; charset=EUC-KR" pageEncoding="EUC-KR"%> <!DOCTYPE html> <form action="" method="post"> <select name="sk"> <option value="u_id">아이디</option> <option value="u_level">권한</option> <option value="u_name">이름</option> <option value="u_email">이메일</option> </select> <input type="text" name="sv"> <input type="submit" value="검색버튼"> </form>
[코드예제] user_search_list.jsp
<%@ page language="java" contentType="text/html; charset=EUC-KR" pageEncoding="EUC-KR"%> <!DOCTYPE html> <%@ page import = "java.sql.DriverManager" %> <%@ page import = "java.sql.Connection" %> <%@ page import = "java.sql.PreparedStatement" %> <%@ page import = "java.sql.ResultSet" %> <%@ page import = "java.sql.SQLException" %> <%@ include file="/usersearch/user_search_form.jsp" %> <br/> <table border="1"> <tr> <td>아이디</td> <td>비번</td> <td>권한</td> <td>이름</td> <td>이메일</td> </tr> <% request.setCharacterEncoding("euc-kr"); String sk = request.getParameter("sk"); String sv = request.getParameter("sv"); Connection conn = null; PreparedStatement pstmt = null; ResultSet rs = null; // 1단계 : 드라이버 로딩 Class.forName("com.mysql.jdbc.Driver"); try { // 2단계 : DB연결 String jdbcDriver = "jdbc:mysql://localhost:3306/dbhooni?" + "useUnicode=true&characterEncoding=euckr"; String dbUser = "idhooni"; String dbPass = "pwhooni"; conn = DriverManager.getConnection(jdbcDriver, dbUser, dbPass); // 3단계 : 쿼리 실행을 위한 객체 생성 if(sk == null && sv == null) { System.out.println("1번 조건 sk, sv 값 null "); pstmt = conn.prepareStatement("SELECT * FROM tb_user"); } else if(sk != null && sv.equals("")) { System.out.println("2번 조건 sk null 아님 sv 값 공백 "); pstmt = conn.prepareStatement("SELECT * FROM tb_user"); } else { System.out.println("3번 조건 sk, sv 값 null 아님"); pstmt = conn.prepareStatement("SELECT * FROM tb_user WHERE " + sk + "=?"); pstmt.setString(1, sv); System.out.println(pstmt); } // 4단계 : 쿼리 실행 rs = pstmt.executeQuery(); while(rs.next()) { %> // html 시작 <tr> <td><%= rs.getString("u_id") %></td> <td><%= rs.getString("u_pw") %></td> <td><%= rs.getString("u_level") %></td> <td><%= rs.getString("u_name") %></td> <td><%= rs.getString("u_email") %></td> </tr> // html 종료 <% } } catch(SQLException ex) { out.println(ex.getMessage()); ex.printStackTrace(); } finally { // 6. 사용한 Statement 종료 if (rs != null) try { rs.close(); } catch(SQLException ex) {} if (pstmt != null) try { pstmt.close(); } catch(SQLException ex) {} // 7. 커넥션 종료 if (conn != null) try { conn.close(); } catch(SQLException ex) {} } %> </table>
04. redirect로 화면 연결
💡
회원가입, 수정, 삭제 후 화면 처리를 user_search_list.jsp 로 리다이렉트 한다.
[코드예제] user_(insert, update, delete)_action.jsp
// 각각 action.jsp 자바코드 맨 마지막 부분에 // user_list.jsp 로 리다이렉트 되어 있는 부분을 // user_search_list.jsp로 리다이렉트로 바꾼다 response.sendRedirect(request.getContextPath() + "/usersearch/user_search_list.jsp");
tag : #DB #JSP #mysql #include #redirect #검색조회 #form #select #option
Uploaded by N2T