01. 익스프레스JS
💡
노드JS에서는 타사 패키지를 설치하기 위한 내장된 매커니즘이 있다.
터미널 → npm 명령
- 노드 패키지 관리자의 약자
- 설치되는 추가 명령인 추가 도구
- 타사 패ㄱ키지 설치하는데 도움이 되는 명령어
npm init → 시스템의 이 표준 폴더를 노드JS관리 프로젝트로 전환
02. 익스프레스 설치
💡
npm install express
🗣
익스프레스 미들웨어
➡️ 요청과 응답 중간에서 동작하는 것
- 요청 오브젝트(request), 응답 오브젝트(response), 미들웨어 함수에 대한 콜백함수
- 모든 코드 실행
- 요청 및 응답 오브젝트에 대한 변경을 실행
- 요청-응답 주기를 종료
- 스택 내의 그 다음 미들웨어를 호출
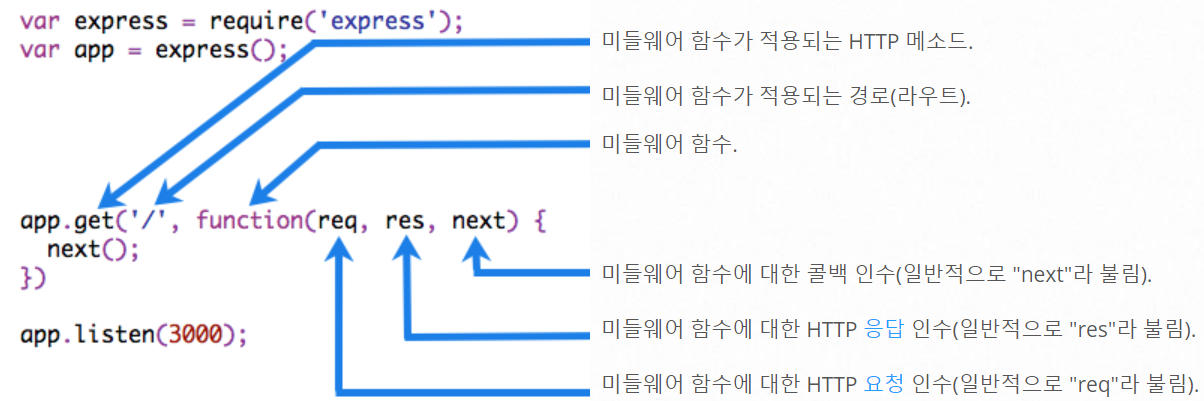
03. 익스프레스 서버 생성
💡
reuire(’express’); → express 패키지 가져오기
- 함수 형태로 반환된다.
- express(); → app 객체를 반환한다.
➡️ app.get(’주소’, function(request, response) {});
- HTTP 요청
- response.send → 응답을 다시 보낼 수 있다.
➡️ app.listen(포트); → 포트넘버 설정
const express = require('express');
const app = express();
app.get('/currenttime', function(req, res) {
res.send('<h1>' + new Date().toISOString() + '</h1>');
});
app.get('/', function(req, res) {
res.send('<h1>Hello World!</h1>');
});
app.listen(3000);
04. 사용자 데이터 구문 분석
💡
form을 이용해서 데이터를 서버측 파일에 저장할 수 있다.
➡️ app.post(’주소’, function(request, response) {});
- HTTP 요청
- request.body → 요청에 첨부된 데이터에 액세스 할 수 있다.
- request.body. → POST 요청에 해당 데이터가 있는 경우에 바디에서 다른 필드에 액세스 할 수 있다.
➡️ app.use()
- 미들웨어 함수를 사용한다.
- 첫번째 인수에 미들웨어가 실행될 경로, 두번째 인수에는 콜백함수가 들어간다.(인수가 두개일 경우)
const express = require('express');
const app = express();
app.use(express.urlencoded({extended: false}));
app.get('/', function(req, res) {
res.send('<form action="/store-user" method="POST"><label>Your Name</label><input type="text" name="userName"><button>Submit</button></form>');
});
app.post('/store-user', function(req, res) {
const userName = req.body.userName;
console.log(userName);
res.send('<h1>Username stored!</h1>');
});
app.listen(3000);
05. 파일에 데이터 저장
💡
➡️ require(’fs’); → 파일시스템 패키지 가져오기
- fs.writeFileSync(); : 파일 쓰기
- fs.readFileSync(); : 파일 읽기
➡️ require(’path’); → 경로 구성에 도움이 되는 패키지 가져오기
- path.join([…paths])
ex) path.join(__dirname, ‘data’, ‘users.json’); → ‘/data/users.json’
➡️ JSON.parse() → json 형태를 자바스크립트 형태로 변환
➡️ JSON.stringfy() → 자바스크립트 형태를 json 형태로 변환
// 파일시스템 패키지 가져오기
const fs = require('fs');
// 경로설정에 도움이 되는 패키지
const path = require('path');
// 익스프레스 타사 패키지 가져오기
const express = require('express');
const app = express();
// 오류 무시?
app.use(express.urlencoded({extended: false}));
// get 방식
app.get('/currenttime', function(req, res) {
res.send('<h1>' + new Date().toISOString() + '</h1>');
});
app.get('/', function(req, res) {
res.send('<form action="/store-user" method="POST"><label>Your Name</label><input type="text" name="userName"><button>Submit</button></form>');
});
// post 방식
app.post('/store-user', function(req, res) {
// 요청 메서드 안에 body로 속성에 접근
const userName = req.body.userName;
// 경로 설정
const filePath = path.join(__dirname, 'data', 'users.json');
// 파일 읽은 후 JSON을 자바스크립트 객체로 파싱
const fileData = fs.readFileSync(filePath);
const existingUsers = JSON.parse(fileData);
existingUsers.push(userName);
// 다시 JSON형태로 변환 후 파일에 저장
fs.writeFileSync(filePath, JSON.stringify(existingUsers));
res.send('<h1>Username stored!</h1>');
});
// 포트 설정
app.listen(3000);
06. 파일 데이터 읽기
💡
1. 파일을 열고 데이터를 읽는다.
2. 원시 텍스트에서 자바스크립트 배열로 변환한다.
3. 해당 데이터와 함께 응답을 다시 보낸다.
// 파일을 읽어서 화면에 출력하기
app.get('/user', function(req, res) {
// 경로 설정
const filePath = path.join(__dirname, 'data', 'users.json');
// 파일 읽은 후 JSON을 자바스크립트 객체로 파싱
const fileData = fs.readFileSync(filePath);
const existingUsers = JSON.parse(fileData); // 배열형태로 출력
let responseData = '<ul>';
for(let user of existingUsers) {
responseData += '<li>' + user + '</li>';
}
responseData += '</ul>';
res.send(responseData);
});
07. 노드몬
💡
노드JS에서 서버를 실행 중이면 서버를 종료한 다음
파일에 변경사항을 저장하고 저장할 때마다 node app.js 명령을 반복해서
새로운 서버를 다시 시작해야 했다.
수동으로 다시 시작해야 작업은 되도록이면 피하고 싶은데 피할 수 있는 방법이 있다.
➡️ npm install nodemon —save-dev 설치
- 설치 후 nodemon app.js 로 실행하면 실행이 안된다.
➡️ package.json에서 스크립트 이름을 수정
- “start” : “nodemon app.js” 를 추가한다.
➡️ npm start 로 실행하면 노드몬이 실행된다.
- 수동으로 다시 시작할 필요가 없이 수정되는 코드가 있으면 자동으로 변경사항이 반영된다.
08. 메소드 정리

📌
➡️ set()
- set(’port’, 3000)을 설정해놨다가 필요할 때 get(’port’)를 이용해서 사용할 수 있다.
➡️ 요청-응답주기

Uploaded by N2T